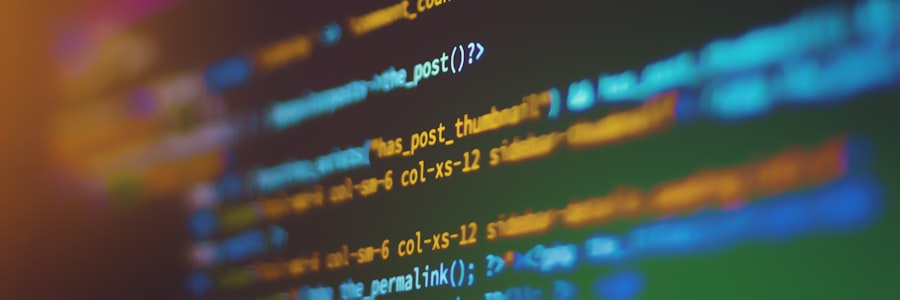
首先需要使用Three.js必须先引入模块
- 你可以选择script的方式快速引用 ``
- 或者通过模块来引入,虽然通过script标签来引入three.js是一个能够快速起步、快速运行的方式,但这种方式对于一些具有较长生命周期的项目来说是有一些缺点。比如:
- 你必须手动获得并在你的项目源代码中包含这个库的一个拷贝
- 更新库的版本是一个手动操作的过程
- 在检查新版本的库时,你的版本差异对比将会被许多行的构建文件给弄乱。
通过npm来安装
Three.js目前已经作为一个npm模块来进行了发布,只需运行”npm install three”就可以使你的项目包含three.js库。
导入这个模块
这次演示使用ES6的方式(import语句)
1 2 3 4
| import * as THREE from 'three';
const scene = new THREE.Scene(); ...
|
或者,如果你希望只导入three.js库中的特定部分,例如Scene:
1 2 3 4
| import { Scene } from 'three';
const scene = new Scene(); ...
|
如何在vue中使用
以下是一个立方体的简单的例子 后续会加上codepen
的示例
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60
| <template> <div> <div id="container"></div> </div> </template> <script> import * as Three from 'three' export default { name: 'Demo', data() { return { camera: null, scene: null, renderer: null, mesh: null } }, methods: { init: function() { let container = document.getElementById('container'); this.camera = new Three.PerspectiveCamera(70, container.clientWidth/container.clientHeight, 0.01, 10); this.camera.position.z = 1; this.scene = new Three.Scene(); let geometry = new Three.BoxGeometry(0.2, 0.2, 0.2); let material = new Three.MeshNormalMaterial(); this.mesh = new Three.Mesh(geometry, material); this.scene.add(this.mesh); this.renderer = new Three.WebGLRenderer({antialias: true}); this.renderer.setSize(container.clientWidth, container.clientHeight); container.appendChild(this.renderer.domElement); }, animate: function() { requestAnimationFrame(this.animate); this.mesh.rotation.x += 0.01; this.mesh.rotation.y += 0.02; this.renderer.render(this.scene, this.camera); } }, mounted() { this.init(); this.animate() } } </script> <style scoped> #container { width: 100vw; height: 100vh; } </style>
|